Scala
'Instance of a class' and 'Object' are synonym in java, but not in scala.
Scala object is a special type of class that can have only one instance.(Scala object represents a singleton class, here we don't call class but call them object.)
Scala doesn't support static members like java. A mechanism to define a static method in Scala, one must place them in an object. Generally we use Scala object for utility methods. So they can be called without even instantiating a class.
Scala Objects can extend Classes, but an object cannot be extended to create a class or another object.
Objects cannot have a public constructor.
Object will only be instantiated on their first use. Next time onwards, same instance would be used. (no use, no instance)
Scala doesn't have 'for' loop.. hmmm.. !!! then, what is 'for' loop syntax in Scala..
Scala 'for' loop is just a syntactical sugar for below control abstractions.
Constructor in Scala
Default Constructor
doesn't take any argument
Primary Constructor
take some argument
Auxiliary Constructor
auxiliary constructors are called with 'this'. auxiliary constructor calls to a previously defined primary constructor.
why do we need 'Auxiliary constructors'
Auxiliary constructors gives flexibility to create instance of class with no / fewer arguments than the PrimaryConstructor.
Apply Methods:
Apply method can be added in Class or Object. But they serve the different purposes when added in class and object.
Apply in Object
Apply in Class
Do you know this, Look at the Return type. How they are changes AnyVal, Any, Int, Option[Int].
Try to relate with above diagram.
Option has 2 subclasses- Some and None
https://docs.scala-lang.org/overviews/collections/overview.html
shows all collections in packagescala.collection
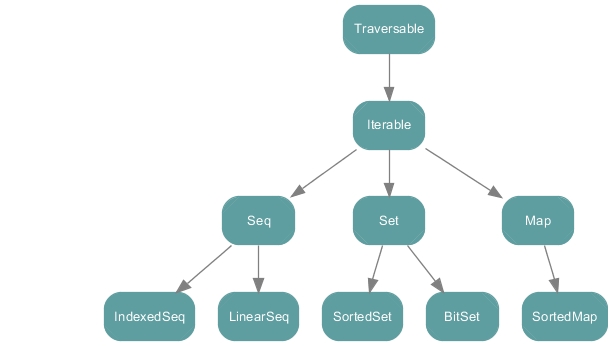
shows all collections in packagescala.collection.immutable
shows all collections in package scala.collection.mutable
The associativity of an operator is determined by the operator’s last character. Operators ending in a colon ‘:’ are right-associative. All other operators are left-associative.
E.g.
Use lift instead of ()
Case classes
Gives you: toString, equals, hashCode, Pattern matching and factory methods
'flatMap' -> to remember the flatMap, apply map first then apply flatten
Null -- is a class.
Nothing -- is a Trait. Its a subtype of everything. But not superclass of anything. There are no instances of Nothing. Nothing has no possible value.
null -- Its an instance of Null- Similar to Java null.
Nil -- Represents an empty List of anything of zero length.so, It refers to List which has no contents.
None -- Used to represent a sensible return value. Just to avoid null pointer exception. Option has 2 subclasses- Some and None. None signifies no result from the method.
Unit– is analogous to a Java method which is declared void. Unit is a subtype of scala.AnyVal. There is only one value of type Unit, ().
A function that doesn't return anything must have the return type Unit. If it were Nothing then the function could not return a result. The only way to exit the function would be by an exception.
java.lang.OutOfMemoryError: Java heap space in REPL
Fixed By:
Starting the Scala REPL with this command to control
Covariant
[+T]
If S is subtype of T, then List[S] is also subtype of List[T]
Contravariant
[-T]
If S is subtype of T, then List[T] is also subtype of List[S]
Invariant
[T]
If S is subtype of T, then List[S] and List[T] are unrelated.
Note: NOTE:- Here List is just used forsimplicity. It can be any valid Scala Type/class etc.
ref : https://docs.scala-lang.org/tour/variances.html
Another Example: trait Function1[-T, +R] from the Scala standard library. Function1 represents a function with one argument, where the first type parameter T represents the argument type, and the second type parameter R represents the return type. Function1 is contravariant over its argument type, and covariant over its return type.
S <: T --- S is a subtype of T
<: T is an upper bound of the type parameter S, it means that S can be instantiated only to types that conform to T.
S >: T --- S is a supertype of T, or T is a subtype of S
S >: T1 <: T2 --- would restrict S any type on the interval between T1 and T2
call scala repl with -Xprint:typer option
combinators (e.g map)
transformer (e.g. =>)
function composition (e.g. andThen)
Today I see even scala document has cheatsheet, Good to check the link
Last updated